Passport.js: The Ultimate Guide to Authentication in Node.js
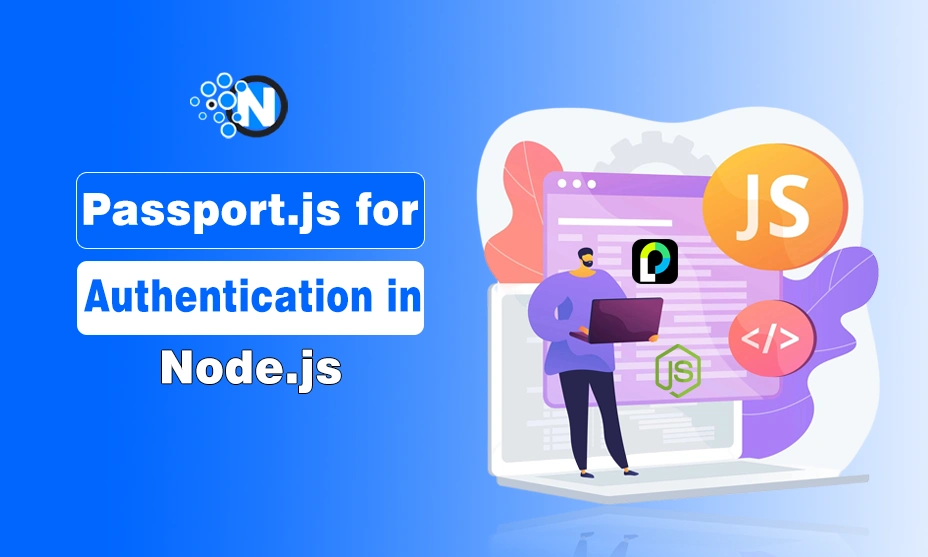
In modern web development, user authentication is one of the most critical aspects of building secure applications. Whether you’re developing a small personal project or a large-scale enterprise application, handling authentication efficiently and securely is essential. One of the most popular authentication libraries for Node.js is Passport.js.
In this blog post, I will share details about Passport.js, its features, use cases, and why it is a preferred choice for handling authentication in Node.js applications.
Let’s start!
What Is Passport.js?
Passport.js is a flexible authentication middleware for Node.js applications. It is designed to authenticate requests, especially useful in applications requiring user login functionality. Unlike other authentication libraries with rigid authentication flows, Passport.js provides a modular approach to authentication, allowing developers to integrate multiple authentication strategies easily.
Passport.js is often used with Express.js, a widely adopted Node.js framework. It supports various authentication mechanisms, including local authentication (username and password), OAuth, and third-party authentication providers like Google, Facebook, X (Twitter), and GitHub.
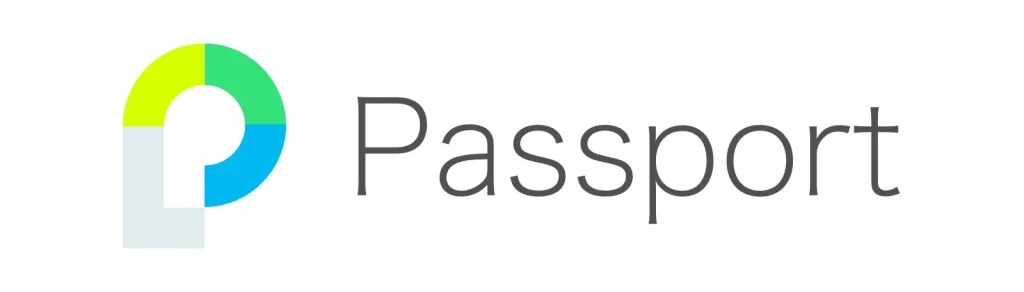
Why Use Passport.js?
There are several reasons why Passport.js is a preferred choice for authentication in Node.js applications:
1. Modular Authentication Strategies
Passport.js supports a wide range of authentication strategies, which are plug-and-play modules that define how users are authenticated. Whether you need a traditional username-password login or OAuth-based authentication, Passport.js makes it easy to integrate multiple authentication mechanisms within a single application.
2. Simplicity and Flexibility
Unlike full-fledged authentication frameworks that enforce a specific structure, it is lightweight and unopinionated. This allows developers to customize authentication workflows according to their application’s requirements.
3. Wide Support for Third-Party Services
With 500+ authentication strategies, Passport.js provides extensive support for third-party login services, including:
- GitHub
- Microsoft Azure
- Apple Sign-In
- Custom OAuth providers
4. Easy Integration with Express.js
Passport.js seamlessly integrates with Express.js applications. It provides middleware functions that simplify handling authentication, user sessions, and redirects.
5. Maintains Session Persistence
For applications that require persistent login sessions, it integrates with session management tools like Express-session, allowing users to remain logged in even after refreshing the page.
Authentication Strategies in Passport.js
A strategy in Passport.js defines how user authentication is handled. Passport.js supports several types of strategies, including:
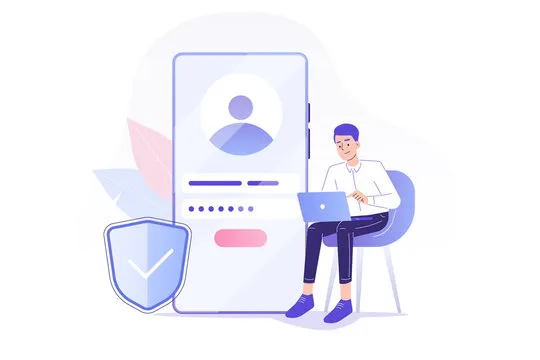
1. Local Strategy
The local strategy allows users to log in using a username and password stored in the application’s database. This is useful for applications that require a traditional login system without third-party authentication.
2. OAuth and OAuth2
OAuth is widely used for authenticating users via third-party providers such as Google, Facebook, and Twitter. It provides built-in support for OAuth and OAuth2 authentication flows, allowing users to log in without creating a separate account for your app.
3. OpenID Connect
OpenID Connect is an authentication protocol built on top of OAuth2. It is commonly used in enterprise applications and supports identity verification from providers like Microsoft, Google, and Apple.
4. JWT (JSON Web Token) Strategy
For stateless authentication, developers can use JWT strategy to authenticate API requests. This is commonly used in RESTful and GraphQL APIs, where users authenticate once and receive a token for subsequent requests.
5. Custom Strategies
It also allows developers to create custom authentication strategies. This is useful when integrating with proprietary authentication systems or non-standard login mechanisms.
How Passport.js Works
The working of Passport.js follows a middleware-based approach:
1. User Requests Authentication
When a user attempts to log in, the request is sent to the server.
2. Passport.js Processes the Request
Passport.js checks if a valid authentication strategy exists for handling the request. If multiple strategies are configured, it determines the appropriate one based on the request type.
3. Authentication Logic Executes
The authentication strategy verifies the user’s credentials. For example:
- In a local strategy, the username and password are validated against the database.
- In an OAuth strategy, Passport.js redirects the user to a third-party provider (e.g., Google) for authentication.
4. User is Authenticated
If authentication is successful, it creates a session or generates a token (depending on the strategy). The user is then redirected to the appropriate page.
5. Accessing Protected Routes
Once authenticated, users can access protected routes that require authentication. It ensures that only authenticated users can access these areas.
Using Passport.js in Different Scenarios
1. User Authentication in Web Applications
For web applications that require user login, Passport.js provides a seamless way to authenticate users with username-password login or OAuth-based authentication.
2. API Authentication
For REST APIs, Passport.js can be used with JWT-based authentication, allowing users to authenticate via tokens instead of sessions.
3. Single Sign-On (SSO)
SSO enables users to log in once and gain access to multiple applications. Passport.js supports OAuth-based SSO, allowing integration with enterprise identity providers like Okta, Auth0, and Azure Active Directory.
4. Social Login Integration
Many modern apps offer social login options such as Google, Facebook, and X (Twitter) login. It simplifies this process by handling OAuth authentication flows for these providers.
Challenges and Considerations
While Passport.js is a powerful authentication library, there are some challenges to consider:
1. Complex Configuration
Setting up authentication strategies, especially OAuth providers, requires API credentials and configuration, which can be time-consuming.
2. Session Management
Handling sessions can introduce complexity, especially for scalable applications where session storage needs to be distributed across multiple servers.
3. Security Considerations
Authentication involves sensitive user data, so additional security measures like encryption, HTTPS, and secure cookie storage should be implemented.
Alternatives to Passport.js
While Passport.js is a popular choice, there are alternative authentication libraries that developers can consider:
- Auth0 – A managed authentication service that provides SSO, social logins, and multi-factor authentication.
- Firebase Authentication – A Google-backed authentication solution for web and mobile applications.
- NextAuth.js – A powerful authentication solution designed for Next.js applications.
- Clerk – A modern authentication library with built-in support for user management.
Each alternative has its own advantages, depending on the project requirements.
Conclusion
Passport.js is one of the most versatile and widely used authentication libraries for Node.js applications. Its modular design, support for multiple authentication strategies, and seamless integration with Express.js make it an excellent choice for developers.
Whether you’re building a simple user authentication system or integrating multiple login providers, Passport.js offers the flexibility to meet diverse authentication needs.
If you’re working on a Node.js project and need a secure, scalable, and customizable authentication solution, Passport.js is a great place to start!