Selenium WebDriver Architecture: Core Components and Implementation
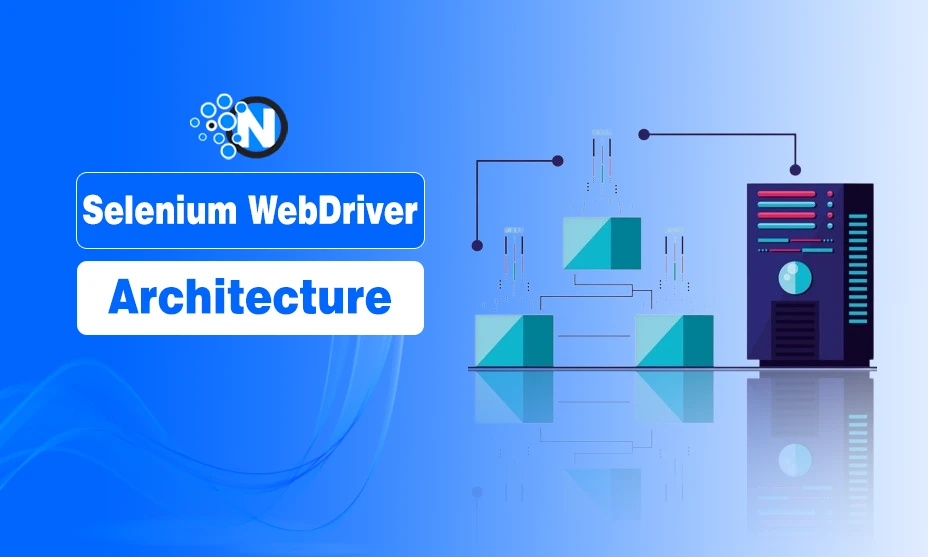
Selenium has become one of the most important parts of modern web app automation testing. The primary reasons are its powerful, flexible, and widely used architecture, along with a vast community of enthusiastic developers and testers.
Selenium WebDriver is the most critical component of the Selenium test suite. Now, various new automation testers and developers might ask, What is Selenium WebDriver?
Do you have this question as well? This is the article for you. Here, we will lay down a detailed overview of Selenium WebDriver’s architecture, its major components, and how you can implement it in real-world automation testing scenarios.
Considering modern testing practices, the testers need to understand these features to create robust automated test cases.
Introduction to Selenium WebDriver
Before we start getting into What is Selenium WebDriver, let us take a step back and understand its basic configuration. It is an open-source tool that will allow the automation of various WebDriver actions.
Being a part of the Selenium test suite, it provides an efficient approach to browser automation. It achieves this goal by interacting with browsers at a deeper level compared to the previously present Selenium Remote Controller. Moreover, the recently released Selenium 4 update has massively improved the capabilities and scope of the WebDriver.
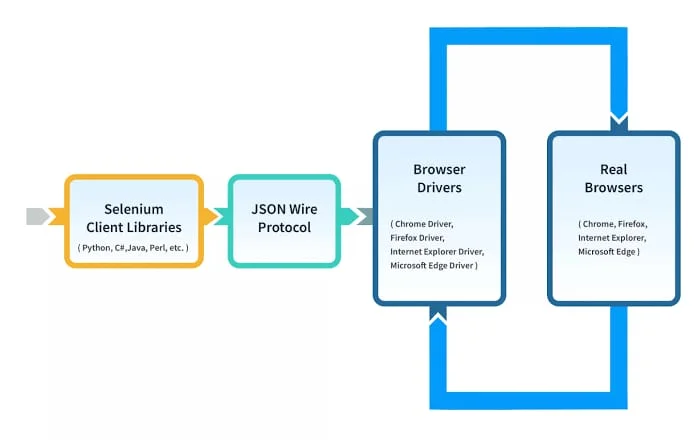
Let us now divert our attention toward some of the major features of Selenium WebDriver that can benefit your automation testing practices:
- The WebDriver will allow you to interact directly with the browser without the need for a server. This is crucial for natively executing the test scripts in the browser window itself.
- The Selenium WebDriver is compatible with all major browsers like Mozilla Firefox, Google Chrome, Safari, and even Microsoft Edge.
- While using this tool, you can write the automation test scripts in any of your preferred programming languages like Java, Python, Ruby and even JavaScript. This approach massively reduces the learning curve that comes with any new software adoption.
- Selenium WebDriver also supports complex actions like drag and drop, clicking, scrolling, and handling multiple popups at the same time.
Selenium WebDriver Architecture
The entire architecture of Selenium WebDriver is built around a client-server model. This architecture comprises multiple layers, which will allow seamless communication between the testing framework and the browser itself.
Let us now divert our attention towards understanding the core components of the similar architecture and their functionality with respect to web automation testing:
Client Library (Language Bindings)
Selenium will natively provide you with multiple client libraries for different programming languages. These libraries will usually contain the WebDriver API, which will, in turn, enable testers to write test scripts in the programming language of their choice.
As we already stated earlier, Selenium supports almost all the currently popular programming languages like Python, JavaScript, C#, and Java.
To further improve our understanding regarding this segment, we have provided a sample automation test script with the Python programming language.
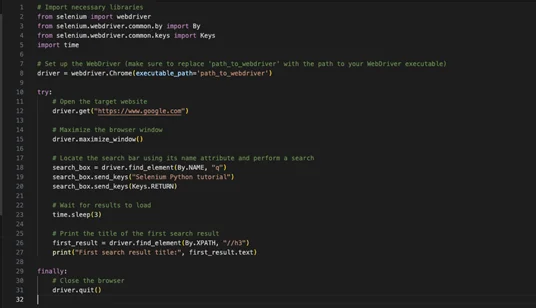
JSON Wire Protocol
The JSON wire protocol will act as a communication bridge between the client library and the browser driver. The primary focus of this component is to standardize the exchange of data in JSON file format.
This component will help you to translate the WebDriver commands like “click to navigate” into HTTP requests.
After this, the browser driver will interpret this request and execute the corresponding action within the browser window. These actions usually include various forms of user interactions on the application that is being tested.
Browser Drivers
For every browser that you are targeting to implement the test case, you will have to install a dedicated driver. This driver will act as a translator between Selenium commands and the browser’s native automation libraries. For example, if you target Google Chrome, you have to use ChromeDriver, GeckoDriver for Mozilla Firefox, EdgeDriver for Microsoft Edge, and SafariDriver for Safari.
The browser drivers will be responsible for executing the following responsibilities during the application testing process:
- They will help you to accept HTTP requests from the client.
- These drivers will also translate these requests into browser-specific commands so that the browser can understand and execute them.
- Finally, it will return the execution status to the client after the test has been successfully run on the web application.
Web Browsers
Web browsers are the final components within the Selenium WebDriver. This is where the automation commands will be executed. Selenium WebDriver supports all major drivers to ensure compatibility across all environments.
Implementation of Selenium WebDriver
To implement the Selenium WebDriver for web automation testing, there are just a few simple steps that you need to follow. To further assist you with this process, we have mentioned all the required steps in the correct order:
Setting Up Selenium WebDriver
The first step involves setting up Selenium WebDriver so that it becomes a part of your web testing environment. To perform this, you have to first download the appropriate driver based on your browser choice. You must also ensure that the driver version matches your browser version as well.
To install the Selenium library, you simply have to use the code that you have attached below:
pip install Selenium
Set Up Browser Drivers
The next step is to set up the browser drivers. For this step, you simply have to place the driver in a directory and add the directory path within the system path.
Writing Your First Test Script
Now that you have finished configuring the Selenium WebDriver and setting it up, all that is left is to write your first automation test script and begin executing it on your target web application. To further help you understand this process, we have mentioned a sample script using the Python programming language:
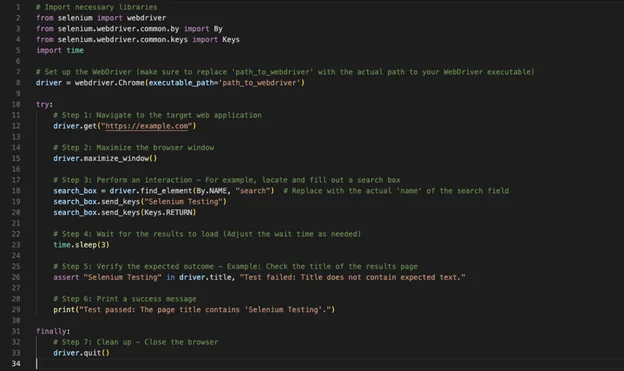
Advanced Interactions
- Selenium will also help you to perform various advanced interactions to further improve your testing process. For example, you can use Selenium for handling various alerts. To perform this process, you simply have to use the code that we have attached below:
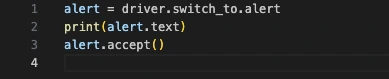
- In case you want to simulate various forms of mouse actions like right click, left click, and scrolling, you simply have to enter the following code in the terminal window during the test execution process.

- If you want to verify the performance of file uploading or data handling on your web application, you can use the attached code during the test execution process:
driver.find_element(By.ID, “file_input”).send_keys(“/path/to/file”)
- Finally, we would suggest the testers explore the possibilities of integrating Selenium with various other tools like PyTest and TestNG. These integrations will allow access to various other advanced features like parameterization and fixtures for tests.
Challenges in Selenium WebDriver Implementation
Now that we have finished understanding the various ways of implementing Selenium WebDriver in your test environment let us go through some of the major challenges that you might face during this implementation process. This information is highly essential to prepare yourself for any unwanted obstacles that will arise during the process:
- Locating dynamic elements in the Selenium web app can be tricky. To resolve this issue, you can use various robust element locators like XPath and CSS selectors.
- While using Selenium WebDriver for implementing web automation testing, you may find it difficult to handle browser-based and JavaScript alerts. To resolve this issue, Selenium WebDriver provides a dedicated method, “driver.switch_to.alert.”
- It would be really complex to ensure that your automation tests run consistently across different browsers and device combinations. The easiest fix to this issue is to regularly update the browser drivers and test on multiple environments. You can also utilize parallel testing to execute these test scripts simultaneously to improve time efficiency.
- In certain scenarios where you might fail to time the test scripts correctly, you will receive flaky test results like false positives and false negatives. The most efficient solution to this problem is the implementation of explicit waits using the “WebDriverwait” method.
Best Practices for Using Selenium WebDriver
We highly advise the testers to incorporate the following best practices while using Selenium WebDriver to improve the quality and efficiency of the testing environment:
- Modern developers have to deal with a lot of dynamic elements. So, to ensure that all these elements are visible and interactable on the application, we suggest the inclusion of explicit waits that are natively available with Selenium. This approach will automatically pause the test execution process until the required element is present and resume it automatically. You can use the following code snippet for this process:

- It is very important to modernize the automation test scripts by breaking them down into reusable functions or classes. This will also help you to easily keep track of the current test execution process.
- We suggest the testers use Page Object Models to organize the locators and actions in separate classes. This approach will not only massively improve the readability of the test scripts but will also help you to efficiently store and reuse them in the coming years.
- The testers can consider using tools like Jenkins or GitHub actions to integrate the Selenium-based automation test scripts with continuous integration and continuous deployment pipelines.
- It is also important for testers to utilize cloud testing platforms like LambdaTest, which can help them integrate advanced practices like AI testing with a remote test lab. LambdaTest is an AI-powered test orchestration and execution platform that lets you perform manual and automation testing at scale with over 3000 real devices, browsers, and OS combinations.
Future of Selenium WebDriver
Finally, let us divert our attention toward some of the most promising upcoming features in Selenium WebDriver:
- The W3C WebDriver protocol will replace the JSON Wire Protocol for standardized communication. This process will further help to streamline all the data transmissions between Selenium WebDriver architecture and the native framework of the browser.
- Selenium will continue to go through major updates, which will improve its mobile testing capabilities. For example, testers will be able to easily integrate mobile testing tools like Appium with Selenium WebDriver.
- With the increasing reliance on artificial intelligence, Selenium will start incorporating various AI testing processes for intelligent element identification.
The Final Verdict
Based on all the factors that we analyzed in this article, we can easily say that understanding the architecture and core components of Selenium WebDriver is very important for scalable and efficient automation tests. Mastering the implementation of Selenium WebDriver, you can ensure robust automation that aligns with modern software development workflows.
Finally, we would like to remind all modern developers and testers that Selenium WebDriver will remain a very important part of the life of an engineer. Moreover, its evolution will promise even greater capabilities for testing in the future.